Demystifying Common Web Server Vulnerabilities: SQLi and XSS Explained
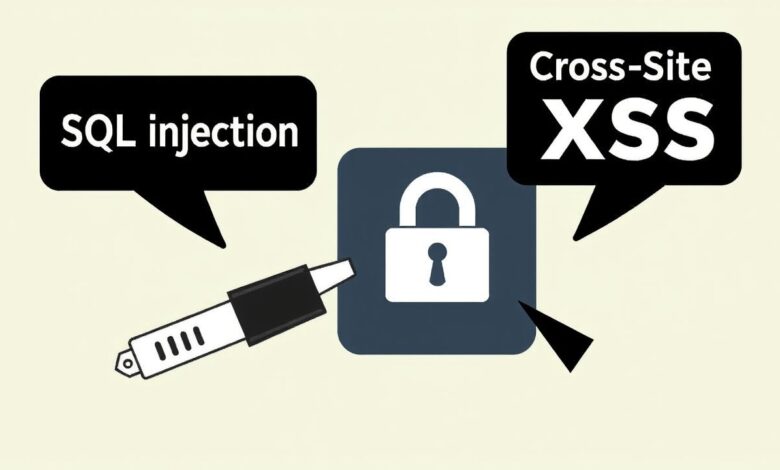
Running a web server, whether it’s for a personal project, a small business website, or a complex application, comes with significant responsibility. One of the most crucial aspects of managing a server is ensuring its security. Failing to protect your server leaves it vulnerable to attacks that can compromise data, disrupt services, and damage your reputation. Among the most prevalent threats are Common Web Server Vulnerabilities, particularly SQL Injection (SQLi) and Cross-Site Scripting (XSS). Understanding these flaws is the first step towards defending against them.
In this post, we’ll break down what SQLi and XSS are, how they work in simple terms, their potential impact, and, most importantly, how you can protect your server from these common attacks.
What is SQL Injection (SQLi)?
SQL Injection, or SQLi, is a code injection technique used to attack data-driven applications. Think of it as an attacker trying to trick your application’s database into executing malicious commands. It occurs when an application builds SQL queries using user input that hasn’t been properly checked or filtered.
How SQLi Works Simply
Imagine a website login form asking for your username and password. When you submit this form, the web application likely creates a SQL query to check if the username and password match a record in the database. A typical query might look something like this (simplified):
“`sql
SELECT user_id FROM users WHERE username = ‘your_username’ AND password = ‘your_password’;
“`
A SQLi vulnerability exists if the application directly inserts your input into this query string without cleaning it. An attacker, instead of typing a username, might input something like `’ OR ‘1’=’1`. If the application is vulnerable, the query becomes:
“`sql
SELECT user_id FROM users WHERE username = ” OR ‘1’=’1′ AND password = ‘attacker_password’;
“`
Since `’1’=’1’` is always true, this modified query might allow the attacker to bypass the username/password check and log in successfully, potentially as the first user in the database (often an administrator).
This is a basic example, but attackers can use SQLi to perform much more sophisticated actions, such as:
- Reading sensitive data from the database.
- Modifying or deleting data.
- Executing administrative operations on the database.
- In some cases, issuing commands to the operating system running the database server.
SQL Injection attacks are incredibly dangerous because they target the heart of many applications: the data. The Open Web Application Security Project (OWASP), a leading authority on web application security, consistently ranks Injection flaws, including SQLi, among the most critical security risks. Historically, SQL Injection has been a known issue since the late 1990s and was ranked as the most critical web application vulnerability in the OWASP Top 10 list in 2013. Even as of 2021, injection vulnerabilities were detected in a vast majority of analyzed applications, highlighting their continued prevalence.
[Hint: Insert image/video illustrating a simplified SQL injection attack]What is Cross-Site Scripting (XSS)?
Cross-Site Scripting, or XSS, is another widespread injection vulnerability, but it targets the users of the vulnerable application rather than the server’s database directly. XSS allows attackers to inject malicious client-side scripts, typically JavaScript, into web pages viewed by other users.
How XSS Works Simply
XSS vulnerabilities often arise when a web application displays user-provided data on a web page without properly sanitizing or encoding it. For instance, consider a comment section on a blog or a forum post where users can submit content. If the application doesn’t process the input correctly, an attacker could submit a comment containing malicious JavaScript code like ``.
When another user views this comment, their browser executes the injected script because it’s part of the legitimate page content delivered from the trusted website. The malicious script then runs within the security context of the vulnerable website. This trust bypasses browser security mechanisms like the same-origin policy, which normally prevents scripts from one website from accessing data or functionality of another.
There are different types of XSS, the most common being:
- Reflected XSS: The malicious script is reflected off the web server, often via a link containing the payload, and executed in the user’s browser. It’s non-persistent.
- Stored XSS: The malicious script is permanently stored on the target server (e.g., in a database) and delivered to users who access the affected page. This is more dangerous as it doesn’t require a specific crafted link.
- DOM-based XSS: The vulnerability exists in the client-side script itself, rather than the server-side code or database.
The Impact of XSS
Once an attacker can execute arbitrary JavaScript in a user’s browser under the context of the legitimate website, they can:
- Steal session cookies, potentially hijacking the user’s session and logging in as them.
- Deface the website for the viewing user.
- Redirect the user to malicious websites.
- Capture user input (like login credentials typed into forms).
- Perform actions on behalf of the user.
- Spread web worms or other malware.
XSS vulnerabilities have been widely exploited and reported since the 1990s, affecting major platforms like Twitter and Facebook in the past. In 2007, some researchers estimated that as many as 68% of websites might be vulnerable to XSS attacks.
[Hint: Insert image/video illustrating a simplified XSS attack flow]SQLi vs. XSS: Key Differences
While both SQL Injection and Cross-Site Scripting are critical web security vulnerabilities stemming from insufficient handling of untrusted input, their targets and mechanisms differ:
- SQLi: Targets the database on the server-side. The malicious input manipulates database queries.
- XSS: Targets the user’s browser on the client-side. The malicious input injects scripts executed by the browser.
Understanding this distinction is key to implementing the right defenses. SQLi focuses on protecting your database interactions, while XSS focuses on protecting the data displayed to users and ensuring it cannot be executed as code.
Protecting Your Server from SQLi and XSS
Preventing these common Web Server Vulnerabilities requires diligence in coding practices and server configuration. Here are essential strategies:
- Never Trust User Input: This is the golden rule. All input coming from users (via web forms, URL parameters, cookies, HTTP headers, etc.) must be treated as potentially malicious.
- Use Prepared Statements and Parameterized Queries (for SQLi): Instead of building SQL queries by concatenating strings, use database features like prepared statements or parameterized queries. These separate the SQL code from the user data, ensuring the input is treated only as data and can never be executed as commands. This is the most effective defense against SQLi.
- Implement Input Validation: Validate user input to ensure it conforms to expected formats, types, and lengths. Reject or sanitize anything that doesn’t meet the criteria.
- Implement Output Encoding (for XSS): When displaying user-provided data on a web page, encode it to prevent the browser from interpreting it as HTML or JavaScript code. For example, convert characters like `<` to `<` and `>` to `>`. This ensures the input is rendered as text, not executable code. Different contexts (HTML body, HTML attributes, JavaScript, CSS) require different encoding methods.
- Use Web Application Firewalls (WAFs): A WAF can provide an extra layer of defense by filtering malicious traffic before it reaches your application, helping to block common attack patterns for SQLi and XSS.
- Keep Software Updated: Regularly update your server’s operating system, web server software (like Apache or Nginx), database software, programming languages, and any libraries or frameworks you use. Updates often include patches for known security vulnerabilities.
- Adopt the Principle of Least Privilege: Ensure that your web application connects to the database using credentials that have only the minimum necessary permissions. This limits the damage an attacker can do even if they manage to achieve SQL injection.
- Conduct Security Audits and Testing: Regularly scan your web applications for vulnerabilities and perform penetration testing to identify potential weaknesses before attackers do.
Securing common server applications like your web server and database is paramount to preventing these attacks. For more on securing these critical components, check out our guide: Fortifying Your Digital Fortress: A Comprehensive Guide to Securing Web and Database Servers.
Conclusion
SQL Injection and Cross-Site Scripting remain two of the most significant and widespread Common Web Server Vulnerabilities affecting applications today. While their attack vectors differ – SQLi targets your database, and XSS targets your users via their browsers – both exploit improper handling of user input. By implementing secure coding practices like using parameterized queries for database interactions and proper output encoding for displaying data, along with regular software updates and security testing, you can significantly reduce your risk and protect your web server and its users from these pervasive threats. Stay vigilant and prioritize security in every stage of development and server management.